2x2 Matrix Multiplication In Python
We will implement each operation of matrix using the Python code. Matrix multiplication is an operation that takes two matrices as input and produces single matrix by multiplying rows of the first matrix to the column of the second matrixIn matrix multiplication make sure that the number of rows of the first matrix should be equal to the number of columns of the second matrix.
20 Examples For Numpy Matrix Multiplication Like Geeks
First make sure you have two vectors.
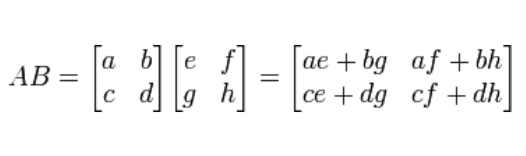
2x2 matrix multiplication in python. We will see both approaches below. To multiply matrix A by matrix B we use the following formula. Here are a couple of ways to implement matrix multiplication in Python.
For j in rangeashape0. Multiplication by a scalar is not allowed use instead. Matrix Multiplication Using Nested List.
A np. A nparray. If X is a n x m matrix and Y is a m x l matrix then XY is defined and has the dimension n x l but YX is not defined.
Matrix Multiplication Vectorized implementation. Note that multiplying a stack of matrices with a vector will result in a stack of. So if you want to multiply the vector A with the matrix B 2x2 this should be C BA where C will be a 2x1 vector.
Numpydot is the dot product of matrix M1 and M2. Second you do res_matrix vec1reshape 10 1 vec2reshape 1 26. Map Reduce Example for Sparse Matrix Multiplication.
Array 0 02 0 2x2 C np. The input files are processed in the mapper such that a key-value pair is emitted with the key being the aggregation key on which we want to aggregate our data. Of columns in matrix 1 no.
Multiplication of two matrices X and Y is defined only if the number of columns in X is equal to the number of rows Y. For smaller matrices we may design nested for loops and find the result. Array 1 10 0 2x2 B np.
Multiplication of two Matrices in Single line using Numpy in Python. The transpose of a matrix is calculated by changing the. In this program we have to use nested for loops to iterate through each row and each column.
To put it in a crude analogy Map Reduce is analogous to the GROUP BY statement in SQL. To multiply them will you can make use of the numpy dot method. A21 B12 A22 B22.
This can be done by checking if the columns of the first matrix matches the shape of the rows in the second matrix. Matrix Multiplication using Nested Loop. After matrix multiplication the prepended 1 is removed.
A nparray12 B nparray3456 C nparray78910 AB npmatmulAB AC npmatmulAC BC npmatmulBC printMatrix A 1x2 printA printMatrix B 2x2 printB printMatrix C 2x2 printC Lets distribute the left multiplication print----- printDistributing left multiplication printMatrix Product AB C 1x2 printnpmatmulABC printMatrix. Of rows in matrix 2. For example vec1shape 10 and vec2shape 26.
A x B. The result of matrix multiplication is a 2x2 matrix. This results in a 22 matrix.
Matrix multiplication is the multiplication of two matrices. For bigger matrices we need some built in functionality in python to tackle this. A11 B12 A12 B22.
Dot B C Out21. In this Python tutorial we will learn how to perform matrix multiplication in Python of any given dimension. If the second argument is 1-D it is promoted to a matrix by appending a 1 to its dimensions.
A11 B11 A12 B21. Import numpy as np create two 2x2 matrix a npmatrix1 2 3 4 using array of array b npmatrix5 6 7 8 using array of array result npmatrixnpzeros22 result matrix printA matrix n a printnB matrix n b traditional code for i in rangeashape1. MATRIX MULTIPLICATION in Python.
Resulti j ai j bi j printnManually calculated result n result get the. After matrix multiplication the appended 1 is removed. Map Reduce paradigm is usually used to aggregate data at a large scale.
The following examples illustrate how to multiply a 22 matrix with a 22 matrix using real numbers. R2Number of Rows of the Second Matrix C1Number of Columns of the First Matrix. A matrix is a rectangular 2-dimensional array which stores the data in rows and columns.
A21 B11 A22 B21. In this article we will introduce the Matrix with Python. Multiplying two matrices in Python.
Multiplication of two matrices X and Y is defined only if the number of columns in X is equal to the number of rows Y or else it will lead to an error in the output result. A nparray 123 456 B nparray 123 456 print Matrix A isnA print Matrix A isnB C npmultiply AB print Matrix multiplication of matrix A and B isnC The element-wise matrix multiplication of the given arrays is calculated in the following ways. Res_matrixshape 10 26.
The matrix multiplication between these two will involve three multiplications between corresponding 2D matrices of A and B having shapes 32 and 24 respectively. The first step before doing any matrix multiplication is to check if this operation between the two matrices is actually possible. Numpydot handles the 2D arrays and perform matrix multiplications.
In numpy row vector and column vector are the same thing. Otherwise if you want to multiply AB and B is still the 2x2 matrix you should define A as a 1x2 vector. Array 1 30 2 2x2 np.
The first matrix is a stack of three 2D matrices each of shape 32 and the second matrix is a stack of 3 2D matrices each of shape 24. This can be formulated as. Using Numpy array.
Here is the full tutorial of multiplication of two matrices using a nested loop. We take two matrices of dimension 2x3 and 3x2 rows x columns. Array2 6 0 0.
Please try your approach on IDE first before moving on to the solution. The matrix can store any data type such as number strings expressions etc. Finally you should have.
We use zip in Python. If X is a n X m matrix and Y is a m x 1 matrix then XY is defined and has the dimension n.
Python And C Program To Implement Multiplication Of 2d Array Matrix Multiplication
Program To Multiply Two Matrix By Taking Data From User Geeksforgeeks
Numpy Matrix Multiplication Numpy V1 17 Manual Updated
Multiplying Matrices 2x2 By 2x2 Corbettmaths Youtube
Matrix Multiplication In Numpy Different Types Of Matrix Multiplication
Matrix Multiplication With 1 Mapreduce Step Geeksforgeeks
Linear Algebra Essentials With Numpy Part 2 By Dario Radecic Towards Data Science
Special Kind Of Row By Row Multiplication Of 2 Sparse Matrices In Python Stack Overflow
Python Multiply Two Matrices Javatpoint
Breakthrough Faster Matrix Multiply
A Complete Beginners Guide To Matrix Multiplication For Data Science With Python Numpy By Chris The Data Guy Towards Data Science
Introduction To Matrices And Vectors Multiplication Using Python Numpy
How To Multiply Two Column Matrices Quora
Python Matrix Multiplication Python Program To Perform Matrix Multiplication
Python Matrix Multiplication The Crazy Programmer
Multiplying Matrices 2x2 By 2x1 Corbettmaths Youtube
Python Matrix Transpose Multiplication Numpy Arrays Examples
Matrix Multiplication In R And Python By Jake Huneycutt Medium